As C++ continues to be a foundational language in software development, particularly in systems programming, game development, and applications requiring real-time processing, understanding “C++ interview questions” is crucial for developers seeking to excel in these fields. This guide will help you navigate the complexities of C++ interviews with confidence.
What are C++ Interview Questions?
C++ interview questions assess a candidate’s proficiency in using C++ to solve complex software development problems. These questions typically cover a wide range of topics, including memory management, object-oriented programming principles, algorithms, data structures, and the nuances of the C++ Standard Library.
Most Common C++ Interview Questions
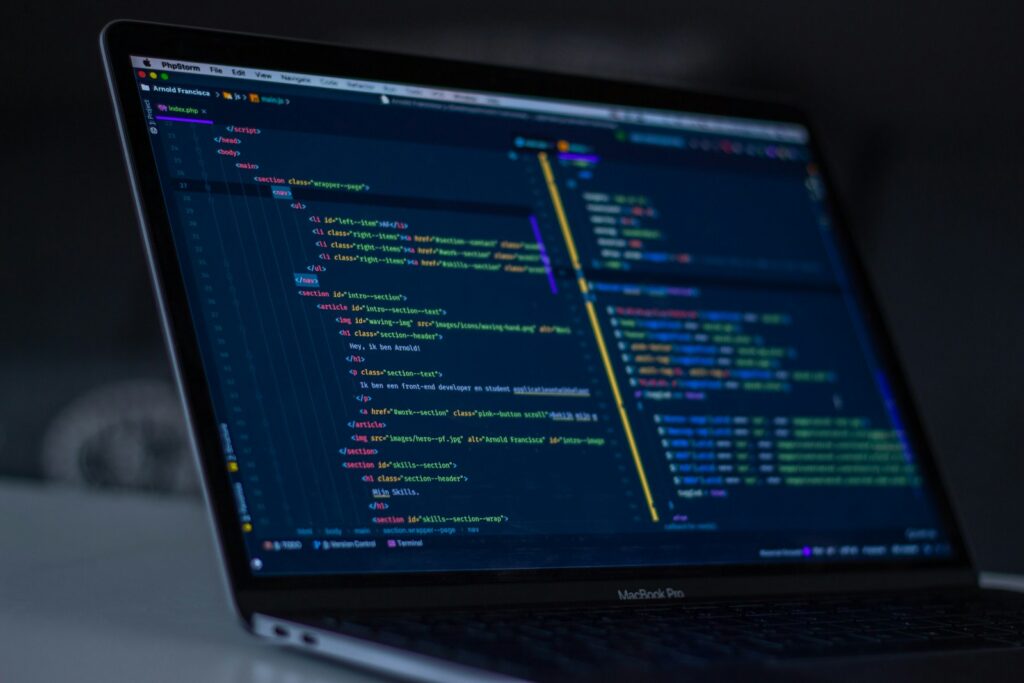
Can you explain the difference between a pointer and a reference in C++?
This question tests your understanding of fundamental C++ concepts that are pivotal for efficient memory management and system-level programming.
Example: “A pointer can hold a null value and can be reassigned to different memory addresses, whereas a reference must be initialized with an existing variable and cannot be changed to reference another variable after initialization.”
Describe how you would implement a singleton design pattern in C++.
Understanding design patterns is essential for writing efficient and maintainable C++ code. This question evaluates your knowledge of software design principles.
Example: “To implement a singleton pattern in C++, I would create a class with a private static pointer to itself and a private constructor. I would then use a static method to control the access to the instance, ensuring only one instance of the class is created.”
What is RAII, and why is it important in C++?
Resource Acquisition Is Initialization (RAII) is a crucial concept in C++ for resource management. This question probes your skills in managing resources such as memory and system handles.
Example: “RAII is a programming paradigm where resource allocation is tied to object lifetime. It’s important in C++ because it ensures that resources are properly released when an object goes out of scope, preventing resource leaks and undefined behaviors.”
How do you handle memory leaks in C++?
Memory management is a key challenge in C++. This question assesses your ability to identify and resolve memory issues.
Example: “To handle memory leaks, I use smart pointers provided by the C++ Standard Library, such as std::unique_ptr and std::shared_ptr, which automatically manage memory and ensure that memory is freed when it’s no longer needed.”
Explain the concept of polymorphism in C++.
Polymorphism is a cornerstone of object-oriented programming. This question tests your understanding of how C++ allows methods to do different things based on the object it is acting upon.
Example: “Polymorphism in C++ allows for methods to have the same name but behave differently on different objects. This can be achieved through either compile-time (function overloading and templates) or run-time (virtual functions) polymorphism.”
What are templates in C++, and how would you use them?
Templates are powerful features for achieving code reuse and flexibility in C++. This question looks at your ability to write generic and type-safe code.
Example: “Templates in C++ allow for writing code that is independent of data type. I use templates to create functions and classes that can operate with any data type, reducing code redundancy and increasing maintenance efficiency, such as implementing generic data structures.”
Can you discuss a time when you optimized C++ code for performance?
Performance optimization is often critical in applications developed in C++. This question allows you to showcase your problem-solving skills and your proficiency in making code efficient.
Example: “In a previous project, I optimized a critical data processing algorithm by reducing unnecessary memory allocation and utilizing move semantics, which significantly improved the execution time by 40%.”
How do you ensure that your C++ code is secure and robust?
Security and robustness are paramount in software development. This question probes your practices for writing secure C++ code.
Example: “I ensure my C++ code is secure by validating all input data, avoiding the use of unsafe functions like strcpy, and regularly using static analysis tools to detect vulnerabilities like buffer overflows.”
Describe the use of lambda expressions in C++.
Lambda expressions enhance the functionality and readability of code. This question tests your knowledge of modern C++ features.
Example: “Lambda expressions in C++ are used to create anonymous functions that can capture variables from their environment. I use them for short snippets of code that are passed as arguments to algorithms or asynchronous callbacks.”
What is the rule of three in C++?
The rule of three relates to C++ class design, crucial for managing resources correctly.
Example: “The rule of three in C++ states that if a class defines one of the following it should probably explicitly define all three: the destructor, copy constructor, and copy assignment operator, to manage resource copying and destruction properly.”
How to Get Prepared for C++ Interview Questions
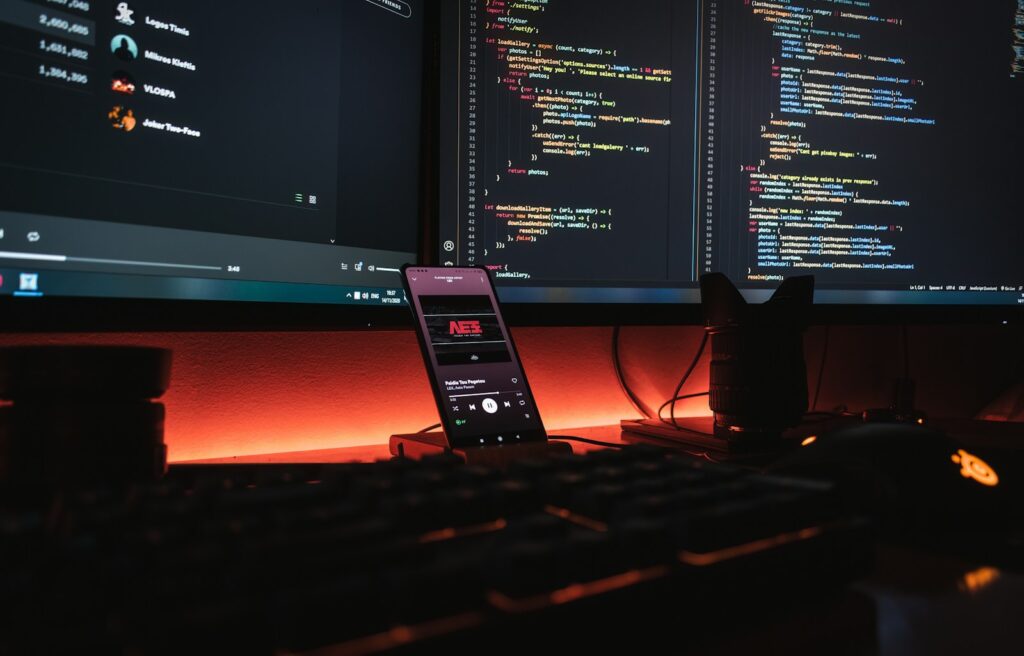
Enhance Your Core C++ Skills
Deepen your understanding of C++ fundamentals, standard library, templates, and new features introduced in the latest C++ standards.
Practice Writing and Refactoring Code
Regularly practice coding to improve your fluency in C++, focusing on writing clean, efficient, and secure code.
Review Advanced Concepts
Study complex topics such as concurrency, memory management, and optimizations to prepare for in-depth discussions.
Participate in Code Reviews
Engage in code reviews with peers to expose yourself to different coding styles and insights which can provide new perspectives and enhance your problem-solving skills.
Special Focus Section: Modern C++ Trends
Discuss the latest trends in C++ development, such as the adoption of C++20 features, the increasing use of C++ in embedded systems, and cross-platform development.
- Key Insight: Explore how modern features like coroutines and modules are changing C++ development paradigms.
- Expert Tip: Highlight best practices for integrating these modern features into existing C++ projects to enhance modularity and performance.
Conclusion
Preparing for C++ interview questions involves a thorough understanding of both fundamental and advanced aspects of the language. By enhancing your knowledge and staying updated on the latest trends, you can demonstrate your expertise and readiness to tackle complex software development challenges with C++ in 2024.
Leave a Reply